Introduction
CamToNES (Camera To Not-Empty Sequence) is command line interface for process security camera videos to get motion and faces.
Rationale
I start writing this program because I need to review hours of security camera videos and that’s sounds really boring and time consuming. The main objetive is to easy the task of reviewing static camera videos applying motion detection through Background subtraction.
Here a incomplete list of things that CamToNES aims to archieve:
-
Have a simple interface to use OpenCV in for extract motion and faces from videos.
-
Provide a simple way to tune the parameters for correctly detect relevant motion.
-
Provide a simple way to real-time detection of movement and faces.
And CamToNES goals are not:
-
To be a fully feature interface to OpenCV.
-
To provide an exhaustive set of tune parameters.
Project Maturity
Since CamToNES is a young project there can be some API breakage.
Dependencies
CamToNES depends on OpenCV 3.0 and python >= 2.7.
Quick Start
The best approach to start to understand how to process a video with CamToNES is using the motion_wizard command.
camtones motion_wizard my_video_file.avi
You will see something like this:
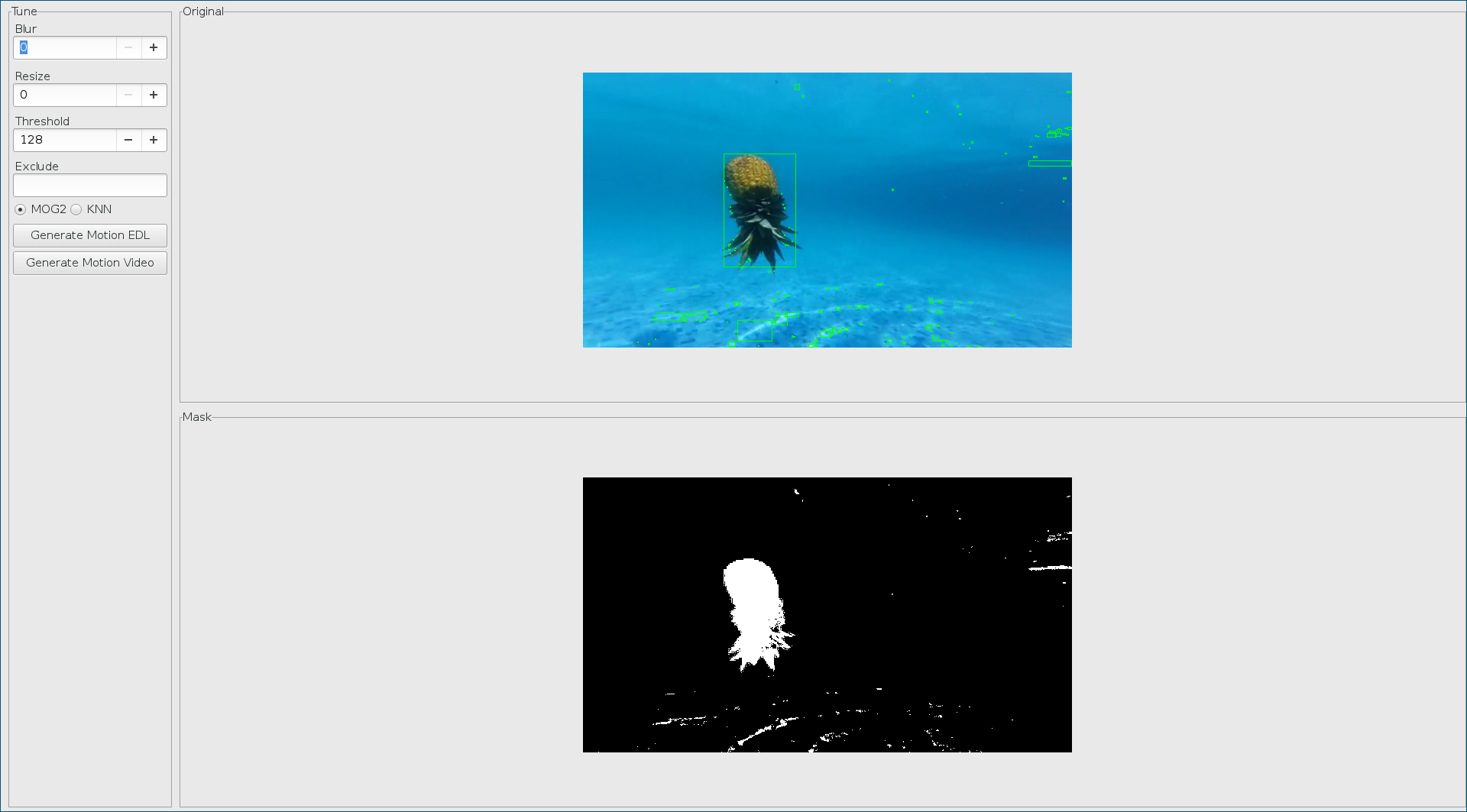
The motion wizard allow you to "tune" the parameters used to extract the motion sections from a video file.
Tunning
You can see the Motion Detection/Extraction Parameters section to understand better the purpose and the behavior of each parameter.
Running extraction
After ensure that you have tunned it correctly, you can start to capture it in a new video file, or an .edl file.
This will have the same behavior if you run the motion_extract or motion_extract_edl commands in the command line, with the parameters defined in the wizard.
Commands
Motion detection
The motion detection reproduce a video or use the video of the webcam, and draw a rect on the detected motion.
For example, you can view motion detected by your webcam with the next command:
camtones motion_detect 0
or the same with a video with:
camtones motion_detect my_video_file.avi
You can tune it with some Motion Detection/Extraction Parameters.
Motion extraction
The motion extraction generate a video containing only the frames that have motion on it.
This command have the --show-time special option that draw a timer that refer the time of the original video.
For example, you can generate a motion only video from a security camera video with the next command:
camtones motion_extract --progress --show-time --exclude "rect.area < 10000" my_video_file.avi my_motion_only_video.avi
The --progress option enable a progress bar.
You can tune it with some Motion Detection/Extraction Parameters.
Motion extraction edl
The motion extraction edl generate a edl file (Edit decision list) containing the information to skip no motion sections of the video.
For example, you can generate a edl file for a security camera video with the next command:
camtones motion_extract_edl --progress --exclude "rect.area < 10000" my_video_file.avi my_video_file.edl
The --progress option enable a progress bar.
After generating the edl file, you can reproduce it with the next mplayer command:
mplayer -edl my_video_file.edl my_video_file.avi
You can tune it with some Motion Detection/Extraction Parameters.
Classifiers
The classifiers command list the included in CamToNES classifiers for face detection/extraction.
Face detection
The motion detection reproduce a video or use the video of the webcam, and draw a rect on the detected faces.
For example, you can view face detected by your webcam with the next command:
camtones face_detect --classifier frontalface_default 0
or the same with a video with
camtones face_detect --classifier frontalface_default my_video_file.avi
You can understand better how face detection works in the section Face Detection/Extraction Parameters.
Face extraction
The face extraction generate a set of files containing faces detected in the video.
For example, you can extract faces from a security camera video with the next command:
mkdir faces
camtones face_extract --progress --classifier frontalface_default my_video_file.avi faces
The --progress option enable a progress bar.
You can understand better how face detection works in the section Face Detection/Extraction Parameters.
Tunning parameters
Motion detection/extraction
The motion extraction/detection get the images of a video, and process it to detect changes between one frame and the previous. This approach, normally, generate a lot of false positives because we are searching for big solid objects in the camera, not small leafs moved by the wind.
For remove this false positive CamToNES process the differences image to remove this false positive. But the false positive can means different things for different videos, this is the reason to need tunning the extraction.
The Process
To filter the image, CamToNES follow the next process. Get a frame from the video, resize it (if the user have define a resize parameter), process it through a background extraction algorithm (MOG2 or KNN). As result, we get a mask of differences between the background and the current frame.
After get the differences mask, we apply to this mask the blur defined by the user.
After blur the mask, we discard all grays, using the threshold provided by the user, this means, that every gray darker than the threshold will be black, and every gray lighter than the threshold will be white.
Every white element remaining in the mask after this process will generate a rectangle result.
This rectangles are filtered by the exclude parameter.
If a frame have a not-excluded rectangle, the frame is a motion frame, else, is a static frame.
Resize
You can resize the image before processing with the --resize option. This is done for process the result faster or for discard some video imperfections.
The value must be a number of pixels of the new width of the frames.
Algorithms
You can defined with the --subtractor parameter the algorithm to use, normally MOG2 and KNN, but depends on your installation. You can see all supported algorithms running motion_detect, motion_extract or motion_extract_edl with the --help option.
The value must be one of the allowed options (normally MOG2 or KNN).
Blur
You can blur the mask to remove camera imperfections or irrelevant small movements with the --blur option.
The value must be a number of pixels.
Threshold
You discard gray zones in the mask with the --threshold option (this normally combined with the blur allow to remove imperfections or small movements). The KNN and MOG2 algorithms detect shadows and assign the gray with value 127 to the shadows.
The value must be a number between 0 and 255.
Exclude
You can exclude result rectangles with the --exclude option. This option receive a python expression that is evaluated to True or False. If the expression evaluate to True, the rectangle is discarded.
The value must be a valid python expression, and you will have access to the variables rect and frame.
The rect variable will have the attributes x, y, width, height and area.
The frame variable will have the attributes width, height and area.
You can use it, for example, to discard any movement with an area smaller than 10000 pixels with the next expression:
rect.area < 10000
Face detection/extraction
The face detection is really a object recognition system based on Haar features. This only means that you can detect objects based on files generated through machine learning. OpenCV comes with some of this files that are included in CamToNES.
You can define your classifier with the parameter --classifier in the face_detect and face_extract commands.
And you can list the available classifiers with the classifiers command:
camtones classifiers
You can use any of this classifiers or use another one using the --classifier option with the file path as parameter.
Contributing
Philosophy
Five most important rules:
-
Beautiful is better than ugly.
-
Explicit is better than implicit.
-
Simple is better than complex.
-
Complex is better than complicated.
-
Readability counts.
All contributions to CamToNES should keep these important rules in mind.
Source Code
CamToNES is open source and can be found on github.
You can clone the public repository with this command:
git clone https://github.com/jespino/catacumba
License
CamToNES is licensed under BSD (2-Clause) license:
Copyright (c) 2015 Jesús Espino <jespinog@gmail.com> All rights reserved. Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.